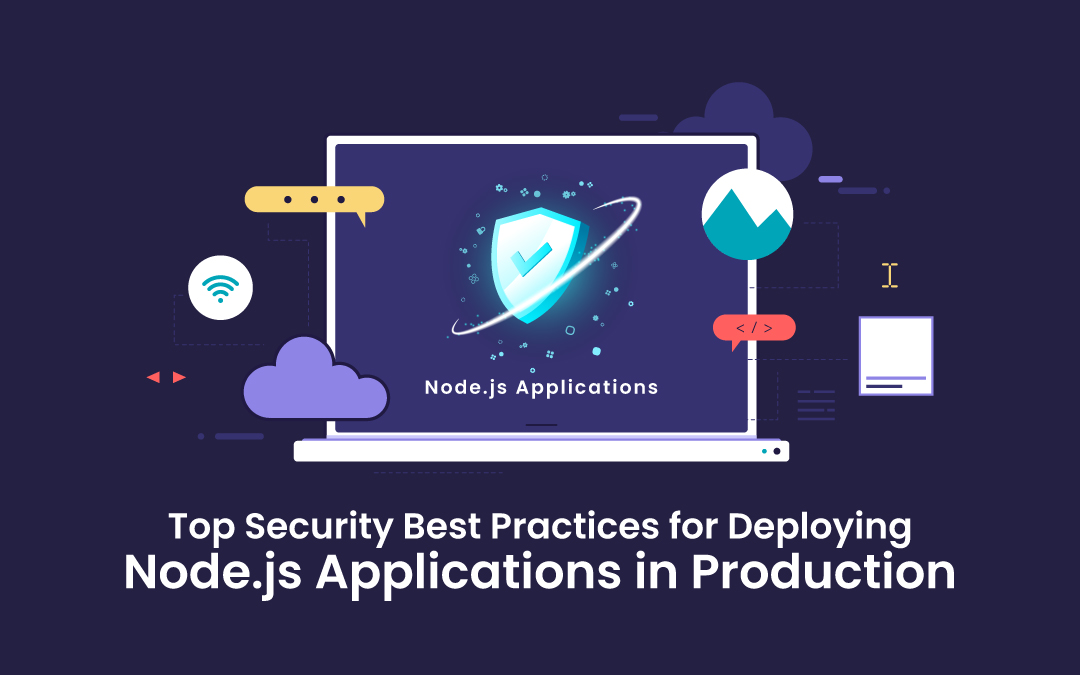
Introduction
Node.js is one of the prominent technologies that developers and even businesses often choose.
According to Statista, 40.8% of developers use Node.js, making it one of the most used web frameworks, as shown in the graph below.
Statista stats on Node.js usage
With its rising popularity in the web development world, Nodejs is often chosen due to its scalability, ease of use, and speed. However, when handling businesses' crucial data, keeping the apps secure is of the utmost importance. And it is true that Nodejs apps are not completely immune to security threats.
That is where securing the node.js apps becomes paramount, especially when they are running in the production environment.
Although it has some built-in security features, it still requires attention to implement the best security practices to develop a secure app.
In this blog, we have compiled 11 best practices for securing your Node.js applications. Let's explore these practices.
Why should you build a secure Node.js application?
Creating a secure Node.js application is important for three main reasons: safeguarding user data, protecting the functionality of the application, and preserving your reputation.
Implementing strong security measures can keep user data safe, prevent application disruptions, and maintain trust with users.
Node.js is powerful because it has access to millions of libraries through the NPM environment. However, many of these NPM packages have security vulnerabilities. This means that your Node.js project is not safe if its dependencies are not. This directly highlights the critical need to secure your Node.js application.
Businesses can leverage the benefits of a secured Node.js application by implementing security measures and hiring skilled Node.js developers.
Best Practices for securing Node.js apps
Securing a Node.js application is imperative to protect data and ensure smooth operations. With increasing cyber threats and other potential attacks, implementing robust security measures is of the utmost importance. To do so, we have compiled some best practices for secure Node.js apps below.
1. Implement security headers
Implementing security headers is essential to ensure the application's security. Without implementing the security headers, the website becomes vulnerable to varied attacks, such as:
- Clickjacking: This technique tricks users into clicking on hidden elements to carry out unintended actions.
- Cross-site scripting: In this, attackers inject malicious code to hijack sessions or steal user data.
- Content injection: This allows unauthorized access and modifications to the website content.
Therefore, the best way to secure the application is to add security headers, which will mitigate this.
One reliable way to do so is to use the "Helmet" library with top frameworks like Express or Fastify.
Adding this helmet automatically adds the essential security headers. For instance, if you use Helmet for Express, you must use the following:
import helmet from "helmet"; app.use(helmet());
In the case of Fastify, you can use the 'fastify-helmet' plugin with the following:
import helmet from "@fastify/helmet"; fastify.register( helmet, { contentSecurityPolicy: false } // Example: disable the `contentSecurityPolicy` middleware );
This will add the essential security headers to the application by default. You can also use some more best practices, such as adding headers like HSTS, X-Frame-Options, X-Content-Type-Options, etc.
2. Error handling and logging
Your apps, servers, and databases can generate errors in production. If these errors are not handled correctly, users might see error messages revealing critical details about the system, like mismatched inputs, network timeouts, and stack traces.
Due to this, attackers may learn about the frameworks used, dependency versions, and insights into your APIs. If exceptions aren't restricted properly, they can bypass security measures.
That is why logging and error handling is required.
To do this, you must thoroughly test your node.js app by sending unexpected data. You can implement the try/catch construct for error handling as shown below:
try { // Code that may throw an error } catch (error) { // Handle the error }
To obtain better insights, you can also use logging tools like Wiston or Pino, which can capture significant events. Examples of some significant events may include:
- Authentication attempts
- Input validation failures
- Attempts to connect with expired or invalid session tokens
- Access control failures
- Any tampering events like an unexpected modification to state data.
3. Never run node.js with root privileges
According to the principle of least privilege, running node.js with root privileges is not recommended.
If you run node.js with root privileges, the vulnerabilities or dependencies can potentially be exploited to gain unauthorized access to the system.
To mitigate this, the best practice is to create a dedicated user for running node.js. This dedicated user is equipped with all the necessary permissions to launch the application.
By restricting attackers to user privileges, we can significantly limit the potential damage they can inflict on the application.
4. Prevent SQL injection
SQL injection is a common attack that takes place when an attacker manipulates the input data passed into an SQL query. In such a scenario, the attackers can forge specific inputs passed to execute arbitrary SQL code. This leads to data breaches and unauthorized access.
To prevent this SQL injection in node.js, you must:
- Employ input sanitization to reject malicious data. This can reduce the risk of SQL injection attacks.
- Use ORM technologies like Sequelize that provide built-in protection against SQL injections.
- Use parameterized queries to separate the SQL code from the user input, which prevents it from being interpreted as part of the query.
To further reduce the risk of SQL injection, consider using Object-Relational Mappers (ORMs) like Sequelize, Knex, TypeORM, or Objection.js. These ORMs have built-in features to interact with databases safely. They hide the complexity of raw SQL queries and automatically protect against SQL injection.
5. Restrict request size
The default request body size limit in Node.js is 5MB. However, it is recommended that this limit be reduced to protect the backend from DoS attacks.
So, to reduce this limit, you can use the body-parser library and configure it as shown below:
const express = require('express'); const bodyParser = require('body-parser'); const app = express(); //Set the request size limit to 1 MB app.use(bodyParser.json({ limit: '1mb' }));
You can adjust the value according to your specific requirements. According to the code above, the request limit is 1 MB, so requests with a larger body than 1MB will be blocked immediately. This prevents the server from allocating resources to process larger requests.
6. Keep dependencies up to date
Keeping dependencies up to date can be a strong tool for securing your Node.js application. Outdated packages often contain vulnerabilities that hackers can exploit. Also, node.js apps usually rely on numerous third-party libraries and packages.
By regularly updating the application's dependencies, you can check for known security vulnerabilities and patch the known vulnerabilities. You can also use tools like 'npm audit,' 'retire.js,' and ‘snyk’ to automatically scan for the vulnerabilities in your dependencies.
This process provides you with the necessary updates and also empowers you to take control of your application's security.
7. Set HTTPS
Use HTTPS everywhere to ensure that all data transmitted between the client and server is encrypted. This will protect the app's data against potential attacks and data interception.
Also, HTTP Strict Transport Security (HSTS) headers should be implemented to enforce HTTPS connections and prevent protocol downgrades.
8. Use Security-Related Linter Plugins
To improve your code's security, you can use linter plugins like eslint-plugin-security. This plugin helps identify vulnerabilities early in the development process, such as unsafe Regular Expressions, improper input validation, and the risky use of eval().
For better results, consider integrating these linting rules with git hooks to enforce security checks before committing code. This helps maintain a secure codebase throughout the development process.
9. Implement role-based access control (RBAC)
A role-based access control (RBAC) is a strong security model that limits access to resources within the application based on user roles. By using RBAC correctly, you can ensure that users only have access to the files and data they need for their roles.
This reduces the risk of unauthorized access and protects the application from potential security breaches. You should define the roles and permissions for different types of users. Also, ensure that they can access only the resources they are authorized to use.
10. Import Built-in Modules Using the 'node:' Protocol
When importing built-in Node.js modules, it's important to always use the "node:" protocol, like this:
import { methodName } from "node:module";
This practice is essential for protecting against typosquatting attacks, where malicious actors may try to trick you into using a compromised package by mimicking the name of a legitimate one.
By explicitly using the node: protocol, you ensure that only official Node.js modules are imported. This reduces the risk of inadvertently including harmful third-party packages.
11. Protect your app from DoS attacks
A Denial of Service (DoS) attack is a type of cyber attack that makes an application inaccessible for its intended purpose. This is typically achieved by overwhelming the application with a high volume of incoming HTTP requests, often generated by a malicious source.
To mitigate such a DoS attack, you must use a reverse proxy to forward and receive requests to the Node app. This can provide load balancing, caching, and IP blacklisting, which reduces the probability of a DoS attack being effective.
Also, limiting the number of total and open sockets per host can help.
Conclusion
We hope this blog has provided you with the best practices for securing node.js applications in production. It is apparent that safeguarding your application is not a one-time task.
It involves implementing strict access control protocols, updating dependencies, and monitoring real-time security events. However, staying on top of everything can be challenging.
That's where partnering with a reliable node.js development company can help. By understanding that maintaining security is an ongoing effort, they can significantly reduce security vulnerabilities while improving the resilience of your node.js applications.
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment